Get Stats Collection Example
View Get Stats Collection example
Get Stats Collection Example
In this tutorial, we will learn how to get stats from an NFT collection with real data using components from MultiversX Design System and MultiversX API.
Requirements
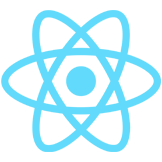
Create a New Next.js Project
See how to create a new project in the official Next.js documentation here: Next.js.
Add Dependencies
We recommend the following dependencies to be added to your package.json file:
{
"name": "Your project",
"version": "1.0.0",
"author": "Author",
"license": "License",
"homepage": "your\*homepage",
"repository": {
"type": "git",
"url": "your_url"
},
"private": true,
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"export": "next export",
"lint": "next lint",
"format": "prettier --write '\*\*/\_.{js,ts,tsx,json}'"
},
"dependencies": {
"next": "^12.2.0",
"react": "17.0.2",
"react-bootstrap": "^2.4.0",
"react-dom": "17.0.2",
"react-router-dom": "^6.3.0",
"sass": "^1.53.0"
},
"devDependencies": {
"@types/jsonwebtoken": "^8.5.8",
"@types/lodash.clonedeep": "^4.5.7",
"eslint": "8.19.0",
"eslint-config-next": "12.2.0",
"eslint-config-prettier": "8.5.0",
"eslint-plugin-prettier": "^4.2.1",
"eslint-plugin-valtio": "0.4.4",
"prettier": "2.7.1",
"typescript": "4.7.4"
}
}
Get Collection from MultiversX API
In your new component created file, you can use the below code to get stats collection data:
import React, {
useEffect,
useState,
useMemo
} from 'react';
const StatsCard = () => {
const [initStats, setInitStats] = useState([]);
const collections = useMemo(
() => [{
ticker: 'NFTIM-4586ac',
name: 'NF-Tim'
},
],
[]
);
// get init api data for collection profile
const getInitStatsPerCollection = (ticker) => {
const promises = ticker.map((data) => {
return fetch(
`https://trust-worker-api.azurewebsites.net/getCollectionProfile/${ticker}` // important to know this API is from XOXNO.com
).then((res) => {
return res.json();
});
});
Promise.all(promises).then((results) => {
setInitStats(results);
});
};
useEffect(() => {
const tickers = collections.map((data) => data['ticker']);
getInitStatsPerCollection(tickers);
}, [collections]);
};
// return ()
export default StatsCard;
Design Stats Card
In the previously created component, you can add the code for the stats card inside the return () statement. This stats card will use the received data to be displayed on our new card:
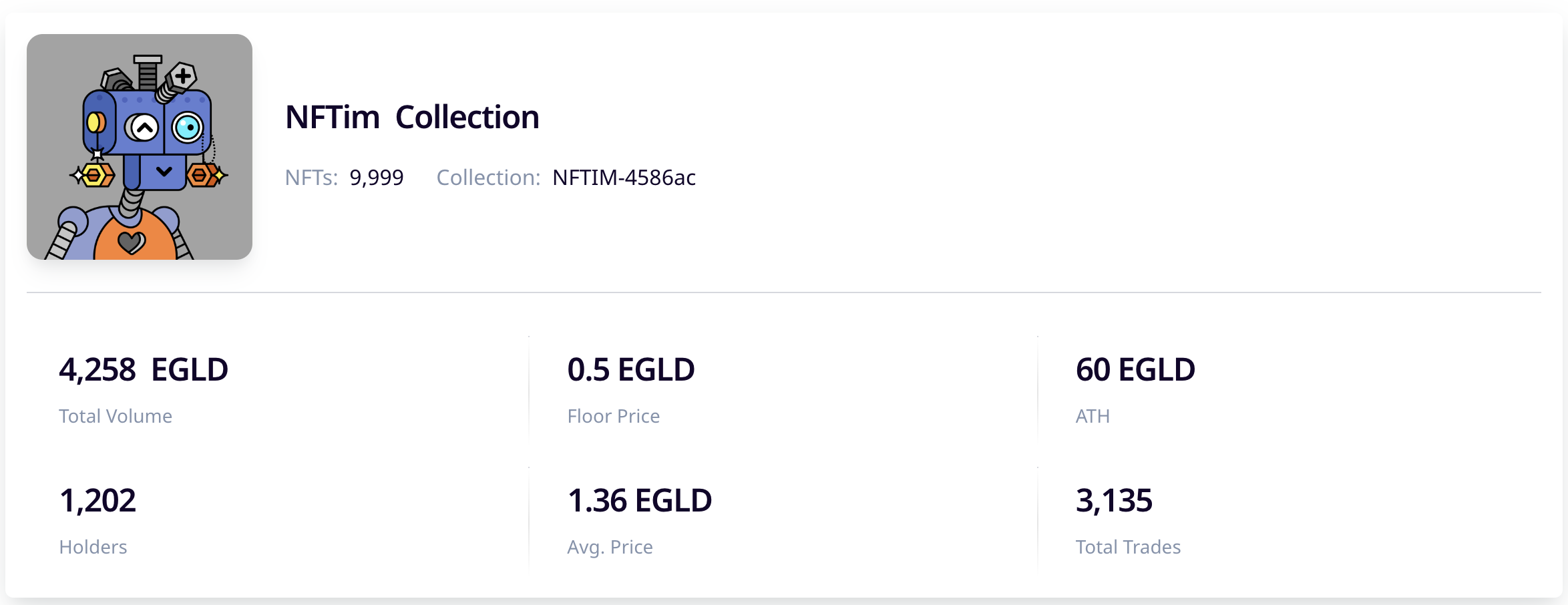
<div className="card card-body mb-4 shadow-lg">
<div className="row">
<div className="col-md-2 text-md-start text-center mb-md-0 mb-4">
<img
className="img-fluid border-radius-lg shadow"
src={
initStats.length > 0
? initStats.imageUrl
: null
}
alt={`image-profile`}
/>
</div>
<div className="col-md-9 d-flex flex-column justify-content-center">
<h4 className="mb-3 text-md-start text-center">
{initStats.length > 0
? initStats.name
: null}
Collection
</h4>
<div className="mb-1 text-md-start text-center">
<span className="me-md-4 me-2 text-secondary font-weight-normal">
NFTs:
<span className="text-dark">
{initStats.length > 0
? initStats.nftCount.toLocaleString()
: null}
</span>
</span>
<span className="text-secondary d-md-inline d-block font-weight-normal">
Collection:
<span className="text-dark">
{initStats.length > 0
? initStats.collectionTicker
: null}
</span>
</span>
</div>
</div>
</div>
<hr className="horizontal dark mt-4 mb-2" />
<div className="row mt-4 gx-2">
<div className="col-md-4 col-6 position-relative">
<div className="card card-body py-2 ps-4 pe-3 shadow-none text-start">
<h4 className="mb-2">
{initStats.length > 0
? initStats.totalEgldVolume.toLocaleString(undefined, {
maximumFractionDigits: 1,
})
: null}
EGLD
</h4>
<h6 className="text-sm text-secondary font-weight-normal mb-1">
Total Volume
</h6>
</div>
<hr className="vertical dark mt-0" />
</div>
<div className="col-md-4 col-6 position-relative">
<div className="card card-body py-2 ps-4 pe-3 shadow-none text-start">
<h4 className="mb-2">
{initStats.length > 0
? initStats.floorEgldPrice
: null}{' '}
EGLD
</h4>
<h6 className="text-sm text-secondary font-weight-normal mb-1">
Floor Price
</h6>
</div>
<hr className="vertical dark d-md-block d-none mt-0" />
</div>
<div className="col-md-4 col-6 position-relative">
<div className="card card-body py-2 ps-4 pe-3 shadow-none text-start">
<h4 className="mb-2">
{initStats.length > 0
? initStats.athEgldPrice
: null}{' '}
EGLD
</h4>
<h6 className="text-sm text-secondary font-weight-normal mb-1">
ATH
</h6>
</div>
</div>
<div className="col-md-4 mt-3 col-6 position-relative">
<div className="card card-body py-2 ps-4 pe-3 shadow-none text-start">
<h4 className="mb-2">
{receivedStatsAPI.getOwnersByCollection.length.toLocaleString()}
</h4>
<h6 className="text-sm text-secondary font-weight-normal mb-1">
Holders
</h6>
</div>
<hr className="vertical dark mt-0" />
</div>
<div className="col-md-4 mt-3 col-6 position-relative">
<div className="card card-body py-2 ps-4 pe-3 shadow-none text-start">
<h4 className="mb-2">
{initStats.length > 0
? initStats.averageEgldPrice.toFixed(2)
: null}{' '}
EGLD
</h4>
<h6 className="text-sm text-secondary font-weight-normal mb-1">
Avg. Price
</h6>
</div>
<hr className="vertical dark mt-0" />
</div>
<div className="col-md-4 col-6 mt-3">
<div className="card card-body py-2 ps-4 pe-3 shadow-none text-start">
<h4 className="mb-2">
{initStats.length > 0
? initStats.totalTrades.toLocaleString()
: null}
</h4>
<h6 className="text-sm text-secondary font-weight-normal mb-1">
Total Trades
</h6>
</div>
</div>
</div>
</div>