Get NFTs Example
View Get NFTs example
Get NFT Example
In this tutorial, we will learn how to create an NFT page with real data using components from MultiversX Design System and MultiversX API.
Requirements
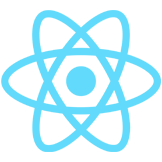
Create a New Next.js Project
See how to create a new project in the official Next.js documentation here: Next.js.
Add Dependencies
We recommend the following dependencies to be added to your package.json file:
{
"name": "Your project",
"version": "1.0.0",
"author": "Author",
"license": "License",
"homepage": "your_homepage",
"repository": {
"type": "git",
"url": "your_url"
},
"private": true,
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"export": "next export",
"lint": "next lint",
"format": "prettier --write '\*_/_.{js,ts,tsx,json}'"
},
"dependencies": {
"next": "^12.2.0",
"react": "17.0.2",
"react-bootstrap": "^2.4.0",
"react-dom": "17.0.2",
"react-router-dom": "^6.3.0",
"sass": "^1.53.0"
},
"devDependencies": {
"@types/jsonwebtoken": "^8.5.8",
"@types/lodash.clonedeep": "^4.5.7",
"eslint": "8.19.0",
"eslint-config-next": "12.2.0",
"eslint-config-prettier": "8.5.0",
"eslint-plugin-prettier": "^4.2.1",
"eslint-plugin-valtio": "0.4.4",
"prettier": "2.7.1",
"typescript": "4.7.4"
}
}
Get Collection from MultiversX API
In your new component created file, you can use the below code to get NFT data:
import React, { useState, useEffect, useMemo } from 'react';
const MyCollection = (props) => {
const ref = React.createRef();
const [loading, setLoading] = useState(true);
const [elrondData, setElrondData] = useState([]);
const [myNFTS, setMyNFTS] = useState([]);
const [address, setAddress] = useState('');
useEffect(() => {
fetch(
`https://api.elrond.com/accounts/${address}/nfts?size=10000`,
{
method: 'GET',
}
)
.then((response) => response.json())
.then((data) => {
setElrondData(Object.entries(data));
})
.catch((err) => {
console.log(err);
});
setTimeout(() => {
setLoading(false);
}, 1000);
}, [address]);
};
Design NFT Card
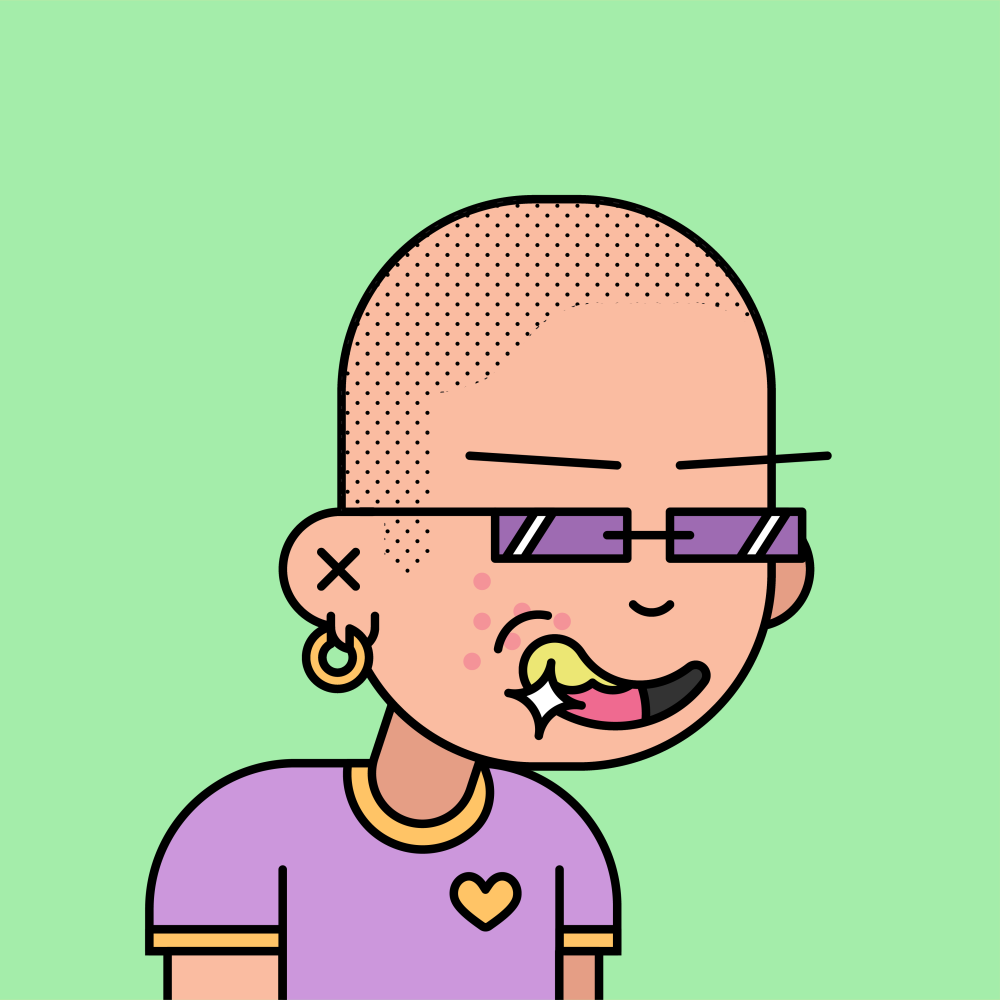
NFT Name
#3
<div className="card card-nft shadow-lg mb-4">
<div className="card-header p-0 mx-3 mt-3 position-relative z-index-1">
<div className="hover-img border-radius-lg">
<img src={`https://media.elrond.com/nfts/assets/${your_CID_collection}/{your_NFT_identifier}.png`} className="img-fluid border-radius-lg" alt="img-0" />
</div>
</div>
<div className="card-body py-3 d-flex justify-content-between">
<h6 className="m-0 font-weight-bold text-capitalize">{your_NFT_name}</h6>
<h6 className="m-0 font-weight-bold">#{your_NFT_identifier}</h6>
</div>
</div>
Add Modal to the NFT Card
In order to see the attributes of the NFT we will need to add a modal to the card.
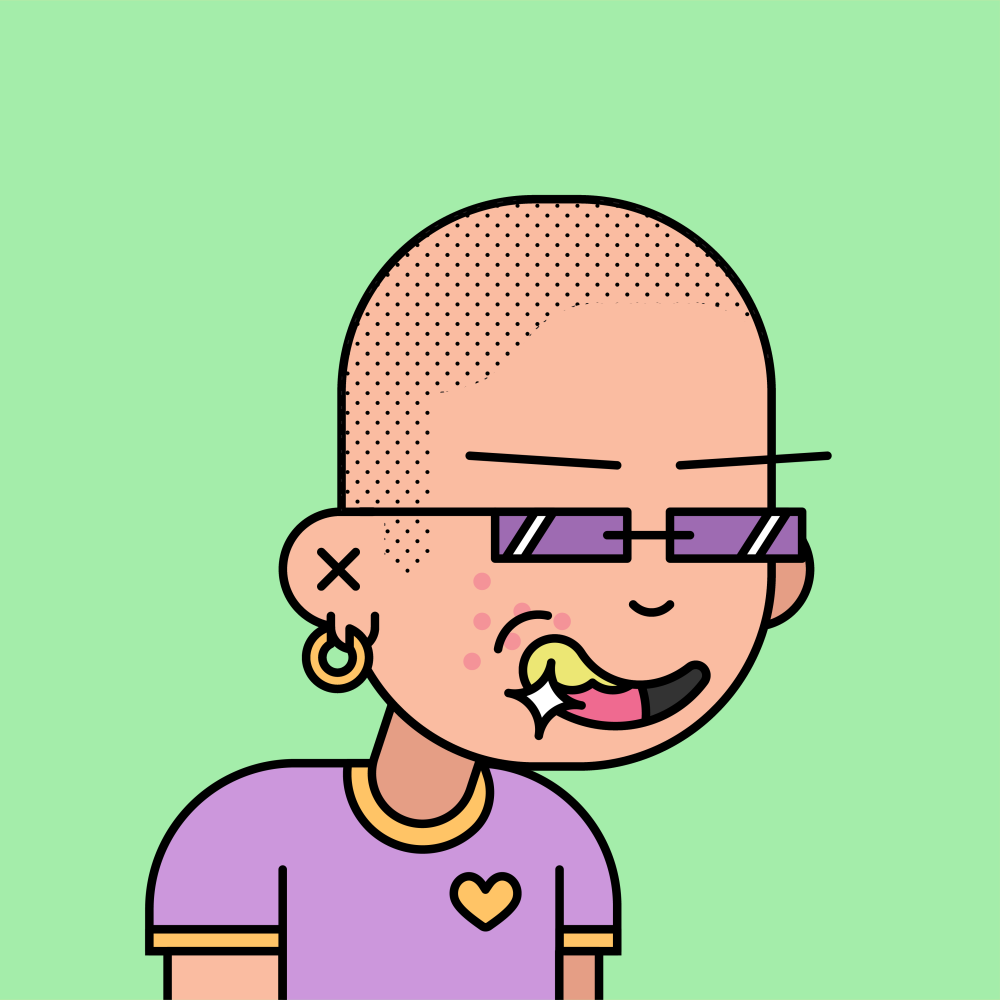
NFT Name
#3
<!-- Card -->
<div className="card card-nft shadow-lg mb-4">
<div className="card-header p-0 mx-3 mt-3 position-relative z-index-1">
<div className="hover-img border-radius-lg">
<img src={`https://media.elrond.com/nfts/assets/${your_CID_collection}/{your_NFT_identifier}.png`} className="img-fluid border-radius-lg" alt="img-0" />
<button
className="btn btn-round btn-hover-nft btn-dark position-absolute mx-6 mx-sm-4 px-2 opacity-0"
onClick={() => {
setModalData([data]);
setModalImage(data?.image);
setModalIsOpen(true);
}}
>
view attributes
</button>
</div>
</div>
<div className="card-body py-3 d-flex justify-content-between">
<h6 className="m-0 font-weight-bold text-capitalize">{your_NFT_name}</h6>
<h6 className="m-0 font-weight-bold">#{your_NFT_identifier}</h6>
</div>
</div>
<!-- Modal -->
<Modal
show={modalIsOpen}
onHide={handleClose}
size="lg"
className="d-flex align-items-center"
>
<Modal.Body className="p-0">
<button
type="button"
className="btn btn-white text-dark shadow-none my-1 top-0 position-absolute z-index-1"
style=
onClick={handleClose}
aria-label="Close"
>
<i className="fas fa-times"></i>
</button>
<div className="card text-center shadow-none">
<div className="card-body">
<div className="row">
<div className="col-sm-5 col-md-6 col-lg-4 mx-sm-auto d-flex align-items-center">
<img
src={modalImage}
className="img-fluid border-radius-lg shadow d-none d-sm-block"
alt=""
/>
</div>
<div className="col-md-12 col-lg-8 mt-4 mt-lg-0">
<div className="row">
<div className="col-md-12 text-start">
<h5 className="text-capitalize mb-3 text-center text-lg-start">
{modalData.map((data, i) => {
return (
<span key={i}>
{data?.attributes[6][1]?.value} #
{data.nonce}
</span>
);
})}
</h5>
</div>
<div className="col-md-6 col-sm-6">
<p className="text-center text-sm-start text-dark text-sm font-weight-normal mb-2">
Attributes
</p>
</div>
<div className="col-md-6 col-sm-6">
<p className="text-center text-sm-end text-dark text-sm font-weight-normal mb-4 mb-lg-2">
Rarity Score:{' '}
<span className="font-weight-bolder">
{modalData.map((data, i) =>
data?.rarity[2][1].toFixed(3)
)}
</span>
</p>
</div>
</div>
{modalData.map((data, i) => (
<div className="row" key={i}>
<div className="col-md-6">
<div className="card card-body py-2 shadow-none border text-start mb-2">
<h6 className="text-xs text-muted font-weight-normal mb-1">
{data?.attributes[0][1]?.trait_type}
</h6>
<h6 className="text-sm m-0">
{data?.attributes[0][1]?.value}
</h6>
</div>
<div className="card card-body py-2 shadow-none border text-start mb-2">
<h6 className="text-xs text-muted font-weight-normal mb-1">
{data?.attributes[1][1]?.trait_type}
</h6>
<h6 className="text-sm m-0">
{data?.attributes[1][1]?.value}
</h6>
</div>
<div className="card card-body py-2 shadow-none border text-start mb-2 mb-lg-0">
<h6 className="text-xs text-muted font-weight-normal mb-1">
{data?.attributes[2][1]?.trait_type}
</h6>
<h6 className="text-sm m-0">
{data?.attributes[2][1]?.value}
</h6>
</div>
</div>
<div className="col-md-6">
<div className="card card-body py-2 shadow-none border text-start mb-2">
<h6 className="text-xs text-muted font-weight-normal mb-1">
{data?.attributes[3][1]?.trait_type}
</h6>
<h6 className="text-sm m-0">
{data?.attributes[3][1]?.value}
</h6>
</div>
<div className="card card-body py-2 shadow-none border text-start mb-2">
<h6 className="text-xs text-muted font-weight-normal mb-1">
{data?.attributes[4][1]?.trait_type}
</h6>
<h6 className="text-sm m-0">
{data?.attributes[4][1]?.value}
</h6>
</div>
<div className="card card-body py-2 shadow-none border text-start">
<h6 className="text-xs text-muted font-weight-normal mb-1">
{data?.attributes[5][1]?.trait_type}
</h6>
<h6 className="text-sm m-0">
{data?.attributes[5][1]?.value}
</h6>
</div>
</div>
</div>
))}
</div>
</div>
</div>
</div>
</Modal.Body>
</Modal>
import Modal from 'react-bootstrap/Modal';
const handleClose = () => setModalIsOpen(false);
const [modalIsOpen, setModalIsOpen] = React.useState(false);
const [modalData, setModalData] = React.useState([]);
const [modalImage, setModalImage] = React.useState([]);
...
See a more complex example here: Elrond My NFTs Collection Dapp by Creative Tim .